- 🌳 Tree-shakeable: Maximize efficiency
- 🪶 Tiny: Just 1.3kb minzipped
- 🚀 Fast: Up to 150% faster than
googlebard
- 📚 Typesafe: Types included out-of-the-box
- 😍 Straightforward API: Learn in minutes
- 💲 Free Forever: Authenticate and use without cost.
- 💨 No dependencies: Uses native
fetch
bard-ai
was built to provide free AI to everyone, through Google Bard.
It's completely free, and takes minimal setup.
Originally based off of acheong08's Python Bard API Code.
Compared to leading JS Bard API googlebard
:
Learn more...
Currently, the most popular JavaScript Bard is GoogleBard by PawanOsman.
However, there are 3 main reasons why bard-ai
is superior:
After being minified and gzipped, bard-ai
is 1.3KB, while google-bard
is 112.8KB (checked with Bundlephobia). That makes bard-ai
~99% smaller!
This library is up to 150% faster, as tested with hyperfine --warmup 1 --runs 3
between bard-ai
and googlebard
1.
googlebard
overcomplicates many things, including importing the cookies to importing and exporting conversations. bard-ai
has been built to make it simple and easy to use.
bard-ai
comes with types, out-of-the-box. Huge thanks to @Al∂haneka for adding this!
bard-ai
uses Node's experimental fetch
function, which is enabled by default now. However, if the environment you are in still doesn't support it, simply install a polyfill, and inject it into global scope.
See how
First, install node-fetch
:
npm install node-fetch
Then, simply include this import and injection before bard-ai
's import.
import fetch from "node-fetch";
globalThis.fetch = fetch;
- Log in to your Google account, and visit Google Bard
- Open the Web Inspector, and go to the "Application" tab.
- Click open the
Cookies
dropdown on the sidebar, under storage, and click on the option that sayshttps://bard.google.com
. - Look for and copy the Cookie labeled
__Secure-1PSID
. Make sure you copy and periods at the end as well.
That's it! Now, when I refer to COOKIE_KEY
in the following document, The __Secure-1PSID
's what I'm referring to.
Warning It is probably a good idea not to commit this
COOKIE_KEY
, though there doesn't seem to be a direct way to exploit it as far as I am concerned.
Go ahead and install bard-ai
with this simple command:
npm install bard-ai
Or, for pnpm
,
pnpm add bard-ai
Always start your code with this:
import Bard from "bard-ai";
await Bard.init("COOKIE_KEY"); // Make sure to replace with your own ID!
That's all the setup you'll need.
If you are doing some form of a request that only needs to ask Bard something once and be done with it (i.e. not continuing in a "chat" form), then askAI()
is the command you are looking for.
Import alongside the entire Bard library like this:
import Bard, { askAI } from "bard-ai";
Or, alternatively, you can use:
import Bard from "bard-ai";
await Bard.askAI("My request");
This second way is more verbose, so I recommend the first way
askAI(message, useJSON)
takes two arguments, the prompt/message you are sending to Bard, and whether or not to return as a JSON, or just a string. See Advanced Usage and Images below to learn how to use useJSON
.
Make sure you use await
on askAI
.
askAI()
returns a string with Bard's response.
import Bard, { askAI } from "bard-ai";
await Bard.init("COOKIE_KEY");
console.log(await askAI("Hello world!"));
If you are doing some form of a project that requires the user to chat and have a dialog with the AI, in which the AI remembers what the user has said, then Bard.Chat()
is the command for you.
Chat()
class is under the Bard library itself, so
import Bard from "bard-ai";
Bard.Chat(IDs)
takes one argument, IDs
, which you can learn more about in the Advanced Usage section below.
Mainly, you use Bard.Chat().ask(message, useJSON)
to ask something to the AI. Bard will remember that message when you ask it again. Chat().ask()
has the same syntax as askAI()
.
You can also use Bard.Chat().export()
to export the IDs
, which you can also read about in the Advanced usage section.
Creating a new Bard.Chat()
instance:
let myChat = new Bard.Chat();
Ask it something...
myChat.ask("Hello there...");
And Bard remembers if you continue!
myChat.ask("What's the last thing I said?");
Here's a similar integrated example:
import Bard from "bard-ai";
await Bard.init("COOKIE_KEY");
let myConversation = new Bard.Chat();
console.log(await myConversation.ask("How are you?"));
console.log(await myConversation.ask("What's the last thing I said?"));
In certain cases, you may need to leave a conversation and continue it later. In this case, you can export a JSON representation of the internal IDs used to keep track of the conversation, and re-import them later.
Let's begin by starting a chat, and saying something.
let myChat = new Bard.Chat();
myChat.ask("What's 1+1?"); // 2
Now, let's export the conversation.
myChat.export();
You should get a JSON similar to this:
{
"conversationID": "YOUR_CONVERSATION_ID",
"responseID": "YOUR_RESPONSE_ID",
"choiceID": "YOUR_CHOICE_ID"
}
Now, we plug it back into a new conversation:
let myContinuedChat = new Bard.Chat({
conversationID: "YOUR_CONVERSATION_ID",
responseID: "YOUR_RESPONSE_ID",
choiceID: "YOUR_CHOICE_ID",
});
myContinuedChat.ask("What's one more than that?"); // Should say 3!
When using useJSON
, you will be returned a JSON with more information. The structure looks like this:
{
content, // string
images: [
{
tag, // string
url // string
}
// array of such objects
],
ids: {
conversationID, // string
responseID, // string
choiceID, // string
_reqID, // stringified integer
}
}
Content is the actual response from the AI, learn about images below, and the IDs are used to export conversations. See above.
New in Bard 2023.05.23, you are now able to see images in Bard answers (for now only in English). bard-ai
has implemented this functionality, and you will now see image links in your Markdown. If you want the image links directly, you can use the useJSON
flag, as shown above. It will give you an array of objects, each with a tag (e.g. [Image of Golden Retriever dog]
) and a URL.
Here's an example of what you may see:
When asked for 5 different images of dogs...
Sure, here are 5 pictures of different dogs:
1. Labrador Retriever

2. Golden Retriever

3. German Shepherd

4. Beagle
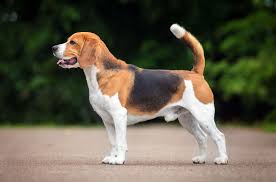
5. Bulldog

Footnotes
-
Run
hyperfine --warmup 1 --runs 3
betweenbard-ai
v1.2.2 andgooglebard
with following code forgooglebard
(in "googlebard.js"):import { Bard } from "googlebard"; let cookies = `__Secure-1PSID=XAgq7axCJiDbtdYALNI-U-L9k_hG-rGEJfkof3UrN93MQk2WHSfP-ZVibAfqTHOxeXuHVw.`; let bot = new Bard(cookies); let response = await bot.ask("Hello world!"); console.log(response);
And following code for
bard-ai
(in "bard-ai.js"):import Bard, { askAI } from "bard-ai"; await Bard.init("MY_KEY"); console.log(await askAI("Hello world!"));
With this benchmark output:
↩Benchmark 1: node bard-ai.js Time (mean ± σ): 6.951 s ± 2.272 s [User: 0.181 s, System: 0.044 s] Range (min … max): 5.333 s … 9.549 s 3 runs Benchmark 2: node googlebard.js Time (mean ± σ): 7.691 s ± 1.029 s [User: 0.389 s, System: 0.073 s] Range (min … max): 6.510 s … 8.394 s 3 runs Summary 'node bard-ai.js' ran 1.11 ± 0.39 times faster than 'node googlebard.js'